Microsoft Entity Framework version 4.0 is a brand new ORM(Object Relational Mapper) from Microsoft. It’s provides now some new features which are not there in the earlier version of Entity framework. Let’s walk through a simple example of a new features which will create a new Entity class based on stored procedure result. We will use same table for this example for which they have used earlier for Linq Binding with Custom Entity.
Below is the table which have simple fields like first name,last name etc.
Let’s insert some data like following.
Below is the stored procedure which I am going to use for this example which will simply fetch data from the table.
Now let’s create Entity model class just like below via Project->Add New Item->Go to Data tab and then select ADO.NET Entity Data Model.
Once you click add a dialog box will appear which will ask for Data Model Contents there are two options Generate From Database and another one is Empty Model. Generate from database will create a Entity model from the ready made database while Empty model enable us to create a model first and then it will allow us to create a database from our model. We are going to use Generate From database for this example. Select Generate From Database like following and click Next.
After click on the next it will ask for database connection string like following where you need to apply connection string for that. I am already having connection string in my web.config so i just selected like below otherwise you need to create one via clicking on new connection. Also you need to specify the connection string name in web.config so it will put a connection string in connection string section with that name.
Once you click next it will fetch the all database objects information like Tables,Views and Stored Procedure like following. Here our purpose is to work with stored procedure so I just selected the stored procedure and selected the GetAllUsers Stored Procedure.
After that it will create a Entity Model class in solution explorer.Now we want to bind the stored procedure with result class so first we need to create function which will call ‘GetAllUser’ stored procedure. To create function we just need to select Our Entity Model class and then go to Model Browser and select the Stored Procedure and right click->Add Function Import like following image.
It will start a new wizard and will go to next step like following image which will have four options 1. None 2. Scalars 3. Complex 4. Entities and there will be a button Get Column information once you click it. It will gather all the column information of stored procedure result set and then click ‘Create New Complex Type’ It will create a new complex type from the gathered column information.
You can also rename that class so I have renamed the class as ‘User Info’ like following.
Now click ok and now it will create function which call the stored procedure and will return Object Result set of Type ‘UserInfo’ which we have just created. Now let’s bind that to a simple grid view to see how its works. So Let’s take a simple grid view like below.
Now Let’s bind that grid view like following from the code behind file of asp.net like following.
Just run it with Ctrl + F5 and below it the output in browser.
That’s it very easy and simple to bind complex type with stored procedure using ADO.NET Entity Framework 4.0. Hope this will help you.. Happy Programming!!!
Below is the table which have simple fields like first name,last name etc.
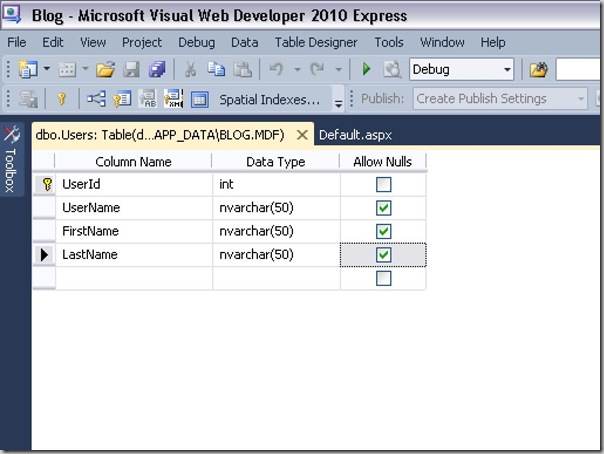
Let’s insert some data like following.

Below is the stored procedure which I am going to use for this example which will simply fetch data from the table.
1 2 3 4 5 6 7 8 9 10 11 12 13 | CREATE PROCEDURE dbo.GetAllUsers AS SET NOCOUNT ON SELECT UserId, UserName, FirstName, LastName, FirstName + ' ' + LastName AS [FullName] FROM dbo.Users |
Now let’s create Entity model class just like below via Project->Add New Item->Go to Data tab and then select ADO.NET Entity Data Model.
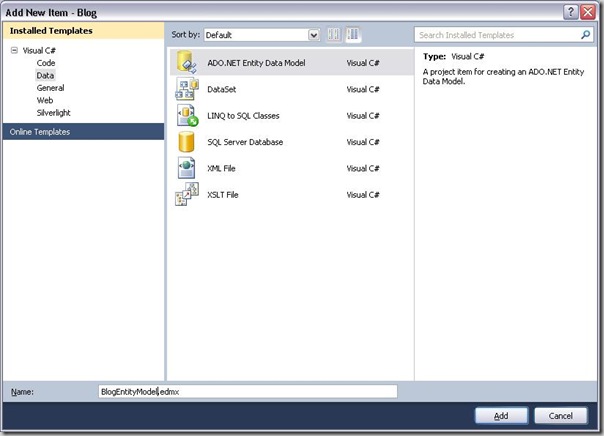
Once you click add a dialog box will appear which will ask for Data Model Contents there are two options Generate From Database and another one is Empty Model. Generate from database will create a Entity model from the ready made database while Empty model enable us to create a model first and then it will allow us to create a database from our model. We are going to use Generate From database for this example. Select Generate From Database like following and click Next.

After click on the next it will ask for database connection string like following where you need to apply connection string for that. I am already having connection string in my web.config so i just selected like below otherwise you need to create one via clicking on new connection. Also you need to specify the connection string name in web.config so it will put a connection string in connection string section with that name.

Once you click next it will fetch the all database objects information like Tables,Views and Stored Procedure like following. Here our purpose is to work with stored procedure so I just selected the stored procedure and selected the GetAllUsers Stored Procedure.
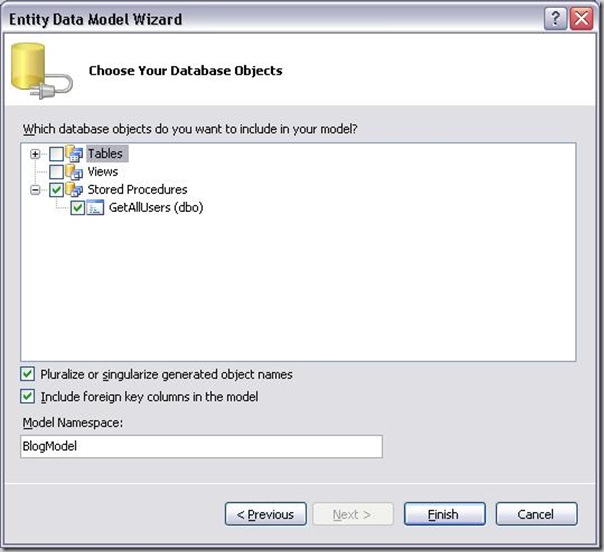
After that it will create a Entity Model class in solution explorer.Now we want to bind the stored procedure with result class so first we need to create function which will call ‘GetAllUser’ stored procedure. To create function we just need to select Our Entity Model class and then go to Model Browser and select the Stored Procedure and right click->Add Function Import like following image.
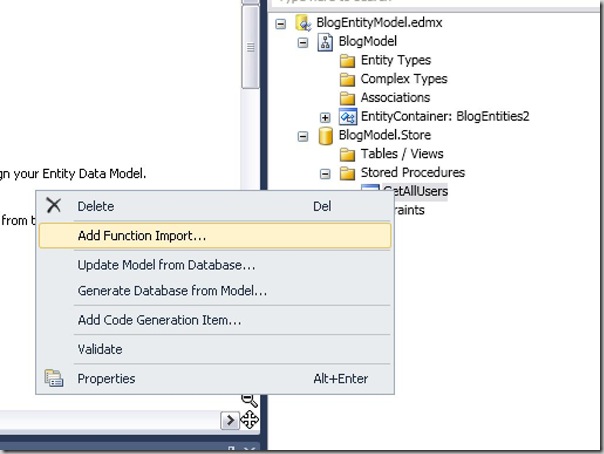
It will start a new wizard and will go to next step like following image which will have four options 1. None 2. Scalars 3. Complex 4. Entities and there will be a button Get Column information once you click it. It will gather all the column information of stored procedure result set and then click ‘Create New Complex Type’ It will create a new complex type from the gathered column information.
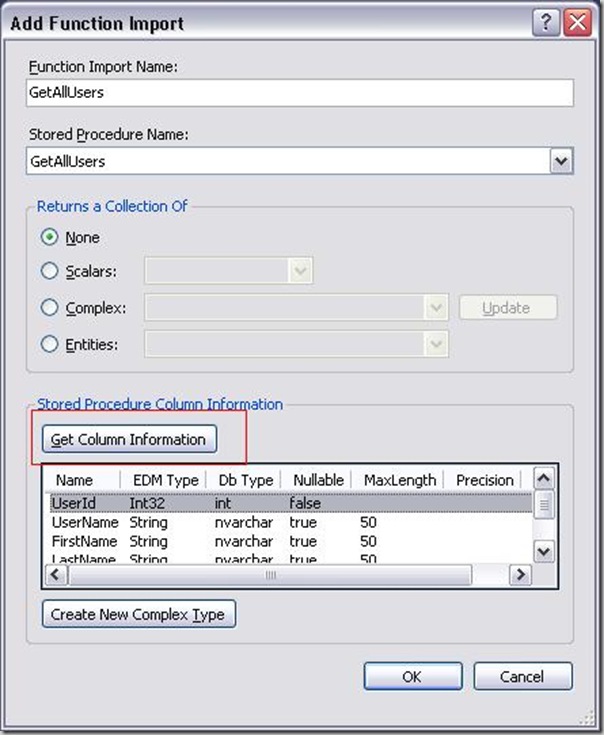
You can also rename that class so I have renamed the class as ‘User Info’ like following.
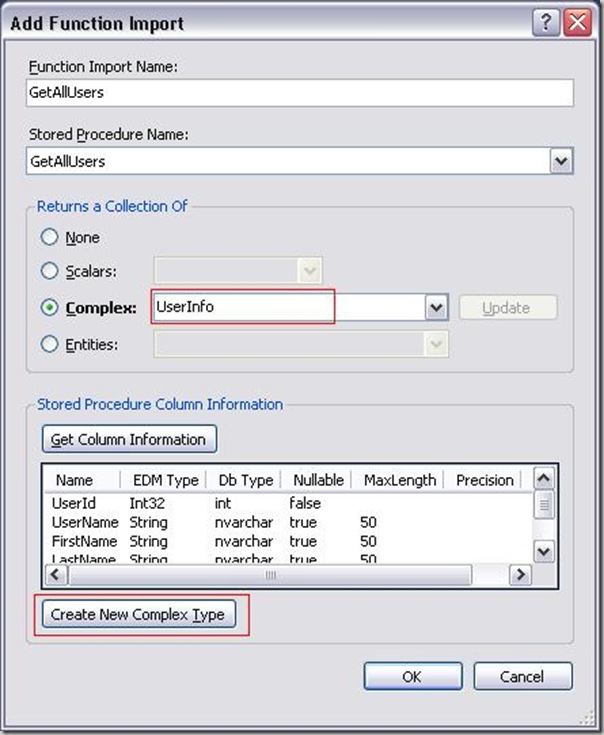
Now click ok and now it will create function which call the stored procedure and will return Object Result set of Type ‘UserInfo’ which we have just created. Now let’s bind that to a simple grid view to see how its works. So Let’s take a simple grid view like below.
1 2 | < asp:GridView ID = "grdUserList" runat = "server" > </ asp:GridView > |
1 2 3 4 5 6 7 8 9 10 11 12 | protected void Page_Load( object sender, EventArgs e) { if (!Page.IsPostBack) { using (BlogEntities myEntity = new BlogEntities()) { grdUserList.DataSource = myEntity.GetAllUsers(); grdUserList.DataBind(); } } } |
Just run it with Ctrl + F5 and below it the output in browser.

That’s it very easy and simple to bind complex type with stored procedure using ADO.NET Entity Framework 4.0. Hope this will help you.. Happy Programming!!!